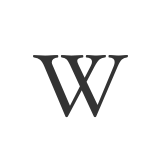
complexity
強制程式中允許的最大循環複雜度
循環複雜度衡量程式碼的線性獨立路徑數。此規則允許設定循環複雜度閾值。
function a(x) {
if (true) {
return x; // 1st path
} else if (false) {
return x+1; // 2nd path
} else {
return 4; // 3rd path
}
}
規則詳細資訊
此規則旨在透過限制程式中允許的循環複雜度來降低程式碼的複雜性。因此,當循環複雜度超過設定的閾值(預設為 20
)時,它會發出警告。
最大值為 2 的錯誤程式碼範例
在遊樂場中開啟
/*eslint complexity: ["error", 2]*/
最大值為 2 的正確程式碼範例
在遊樂場中開啟
/*eslint complexity: ["error", 2]*/
function a(x) {
if (true) {
return x;
} else {
return 4;
}
}
function b() {
foo ||= 1;
}
類別欄位初始化程式和類別靜態區塊是隱含的函式。因此,它們的複雜度是針對每個初始化程式和每個靜態區塊分別計算的,並且不會影響封閉程式碼的複雜度。
最大值為 2 的其他錯誤程式碼範例
在遊樂場中開啟
/*eslint complexity: ["error", 2]*/
class C {
x = ; // this initializer has complexity = 3
}
class D { // this static block has complexity = 3
}
最大值為 2 的其他正確程式碼範例
在遊樂場中開啟
/*eslint complexity: ["error", 2]*/
function foo() { // this function has complexity = 1
class C {
x = a + b; // this initializer has complexity = 1
y = c || d; // this initializer has complexity = 2
z = e && f; // this initializer has complexity = 2
static p = g || h; // this initializer has complexity = 2
static q = i ? j : k; // this initializer has complexity = 2
static { // this static block has complexity = 2
if (foo) {
baz = bar;
}
}
static { // this static block has complexity = 2
qux = baz || quux;
}
}
}
選項
此規則具有數字或物件選項
-
"max"
(預設值:20
)強制執行最大複雜度 -
"variant": "classic" | "modified"
(預設值:"classic"
)要使用的循環複雜度變體
max
使用 max
屬性自訂閾值。
"complexity": ["error", { "max": 2 }]
已棄用: 物件屬性 maximum
已棄用。請改用屬性 max
。
或使用簡寫語法
"complexity": ["error", 2]
variant
要使用的循環複雜度變體
"classic"
(預設值)- 經典 McCabe 循環複雜度"modified"
- 修改後的循環複雜度
修改後的循環複雜度與經典循環複雜度相同,但每個 switch
陳述式只會將複雜度值增加 1
,無論它包含多少個 case
陳述式。
具有 { "max": 3, "variant": "modified" }
選項的此規則的正確程式碼範例
在遊樂場中開啟
/*eslint complexity: ["error", {"max": 3, "variant": "modified"}]*/
function a(x) { // initial modified complexity is 1
switch (x) { // switch statement increases modified complexity by 1
case 1:
1;
break;
case 2:
2;
break;
case 3:
if (x === 'foo') { // if block increases modified complexity by 1
3;
}
break;
default:
4;
}
}
上述函式的經典循環複雜度為 5
,但修改後的循環複雜度僅為 3
。
何時不該使用
如果您無法確定程式碼的適當複雜度限制,最好停用此規則。
相關規則
版本
此規則是在 ESLint v0.0.9 中引入的。
延伸閱讀
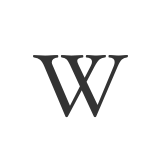
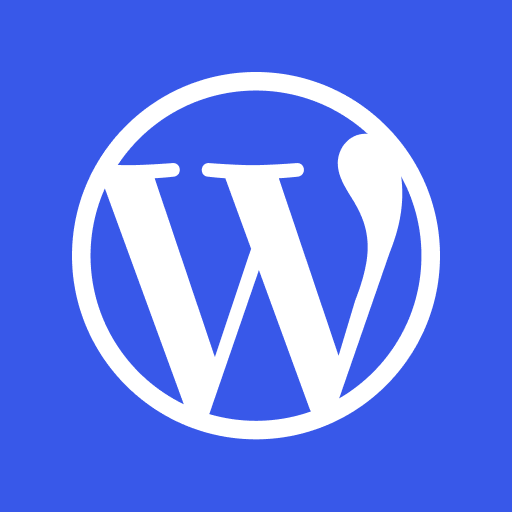
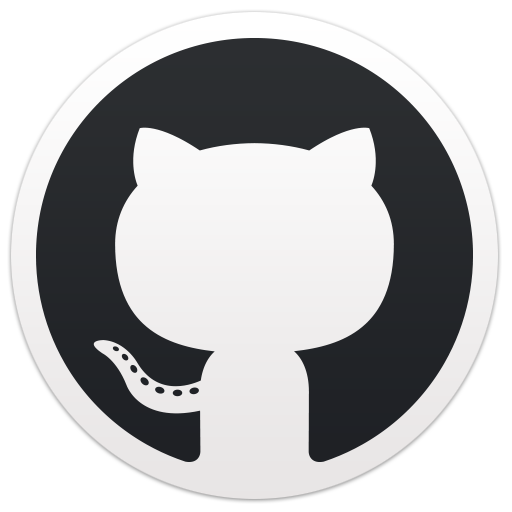